kbone
kbone 项目(GitHub 上的开源项目)实现了一个适配器,该适配器在适配层中模拟浏览器环境,以便为 web 编写的代码可以在小程序中无需更改即可运行。存在一些启动器模板(其中包括 Vue、React 和 Preact),这些模板使来自这些框架的 web 开发者更容易上手。
可以使用 kbone-cli
工具创建新项目。向导会询问使用什么框架来启动项目。下面的代码段显示了 Preact 演示。在下面的代码段中,mp
命令构建小程序,web
命令构建 web 应用,而 build
创建生产 web 应用。
npx kbone-cli init my-app
cd my-app
npm run mp
npm run web
npm run build
下面的代码段显示了一个简单的计数器组件,该组件随后在小程序和 web 应用中同构呈现。仅从 DOM 结构来看,小程序的开销非常大。
import { h, Component } from "preact";
import "./index.css";
class Counter extends Component {
state = { count: 1 };
sub = () => {
this.setState((prevState) => {
return { count: --prevState.count };
});
};
add = () => {
this.setState((prevState) => {
return { count: ++prevState.count };
});
};
clickHandle = () => {
if ("undefined" != typeof wx && wx.getSystemInfoSync) {
wx.navigateTo({
url: "../log/index?id=1",
});
} else {
location.href = "log.html";
}
};
render({}, { count }) {
return (
<div>
<button onClick={this.sub}>-</button>
<span>{count}</span>
<button onClick={this.add}>+</button>
<div onClick={this.clickHandle}>跳转</div>
</div>
);
}
}
export default Counter;
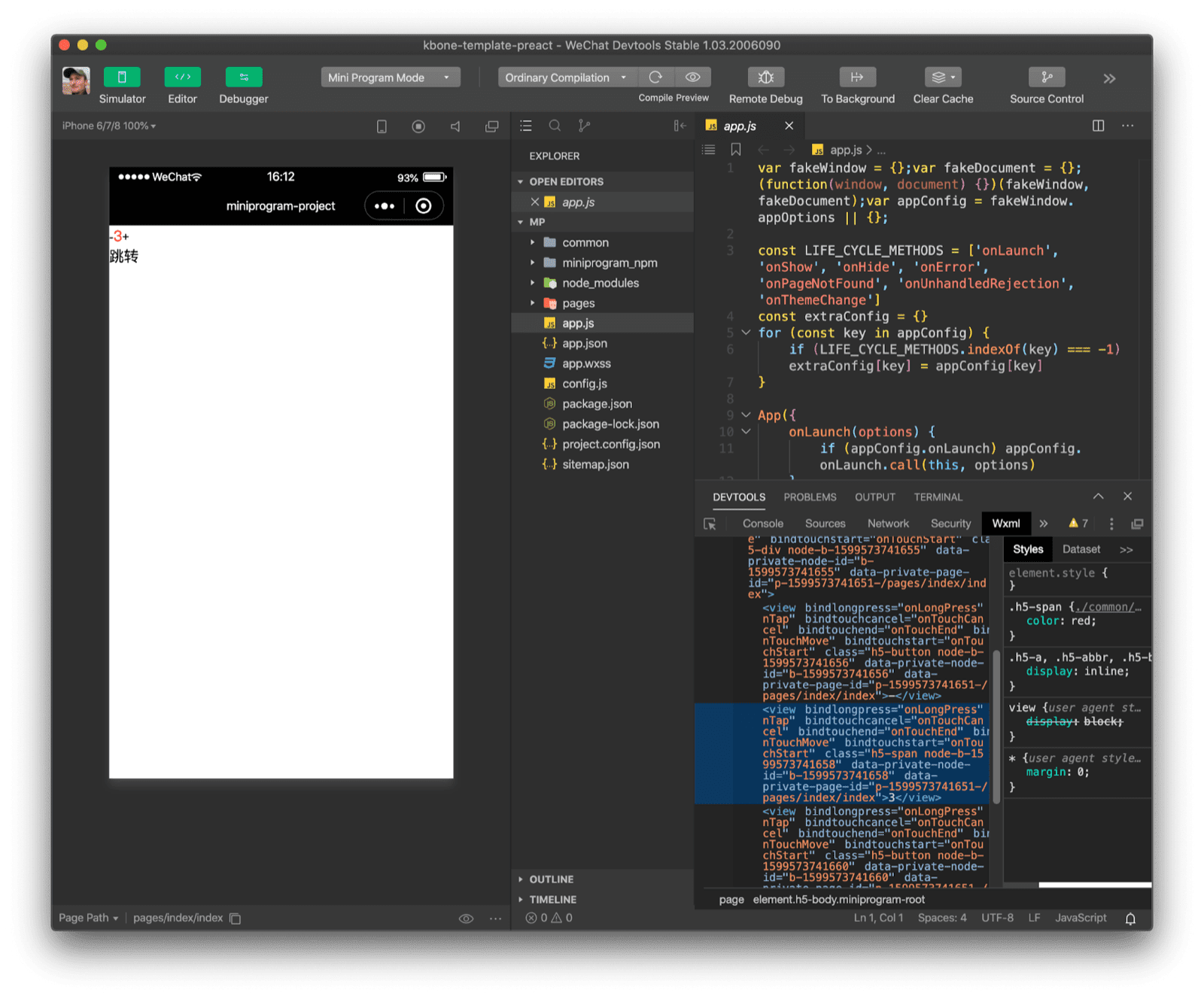
<button>
元素。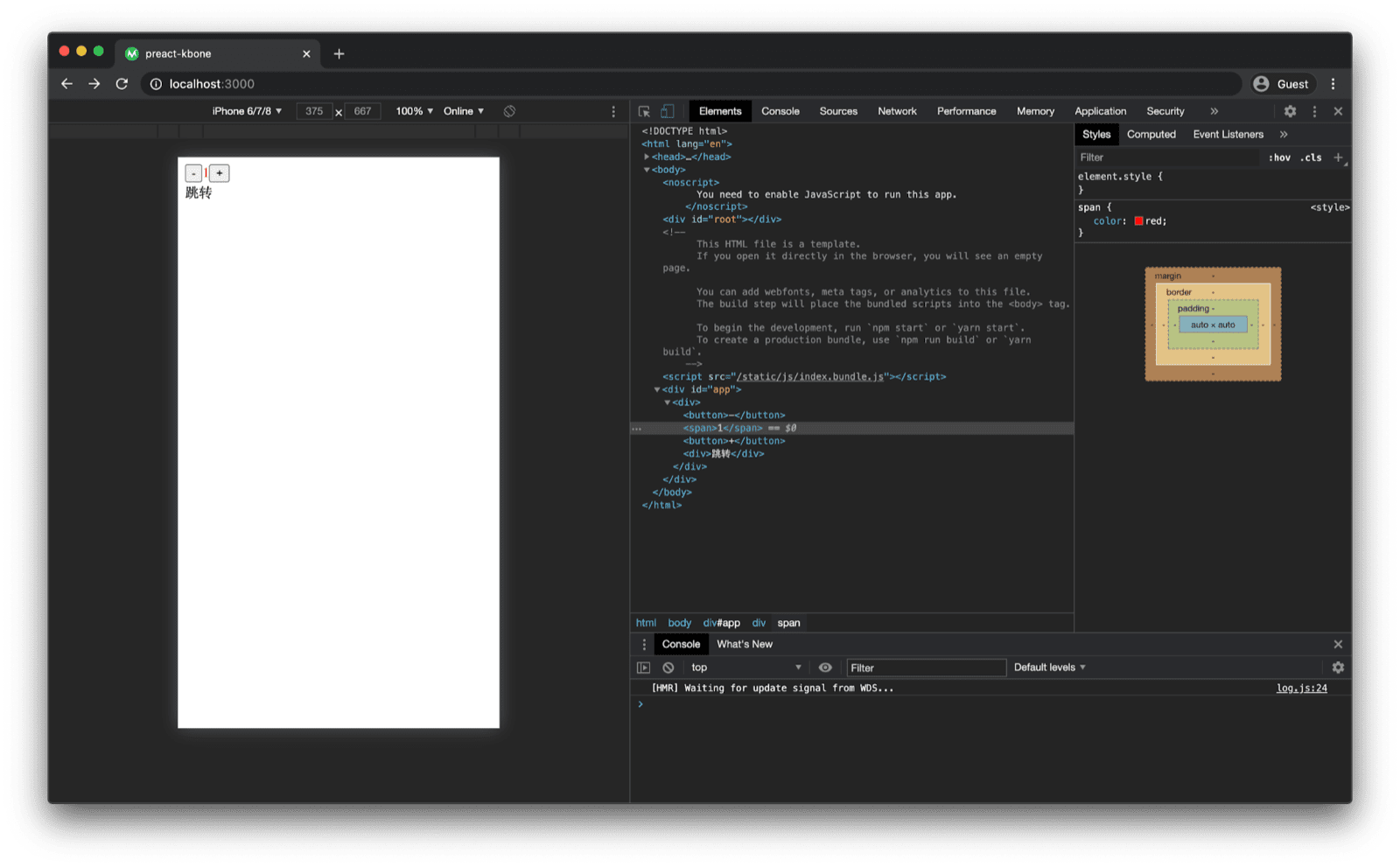
kbone-ui
kbone-ui 项目(GitHub 上的开源项目)是一个 UI 框架,可促进小程序开发以及使用 kbone 进行 Vue.js 开发。kbone-ui 组件模拟了 微信的内置小程序组件 的外观和风格(另请参见上面的组件)。一个直接在浏览器中运行的 演示 让您可以探索可用的组件。
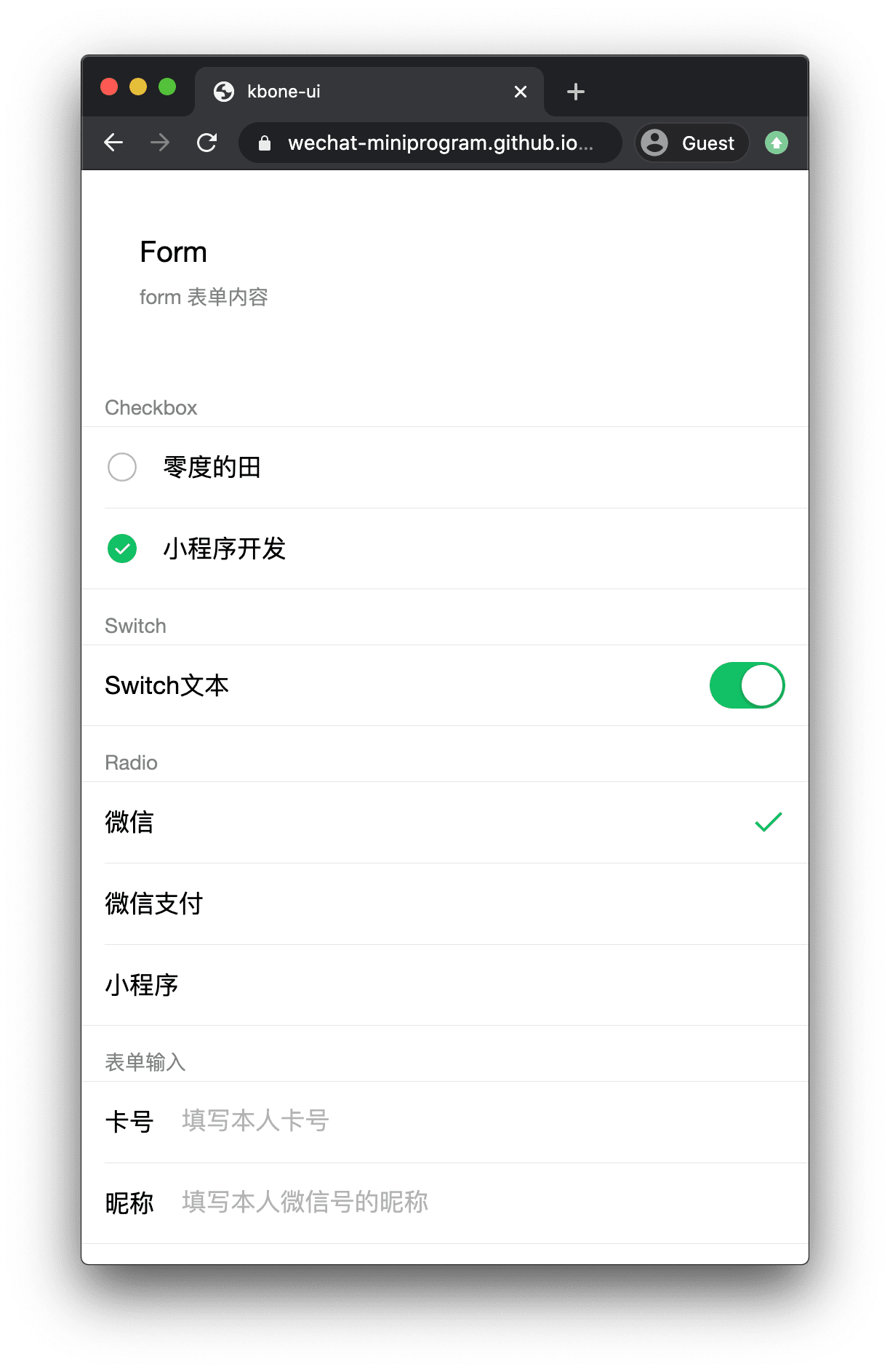
WeUI
WeUI 是一组与微信的默认视觉体验一致的基本样式库。微信官方设计团队为微信内部网页和微信小程序量身定制了设计,使户对使用的感知更加统一。它包括 button
、cell
、dialog
、progress
、toast
、article
、actionsheet
和 icon
等组件。有不同版本的 WeUI 可用,例如用于使用 WXSS 样式化的微信小程序的 weui-wxss(请参阅上面的样式)、用于 web 应用的 weui.js 和用于微信 React 组件的 react-weui。
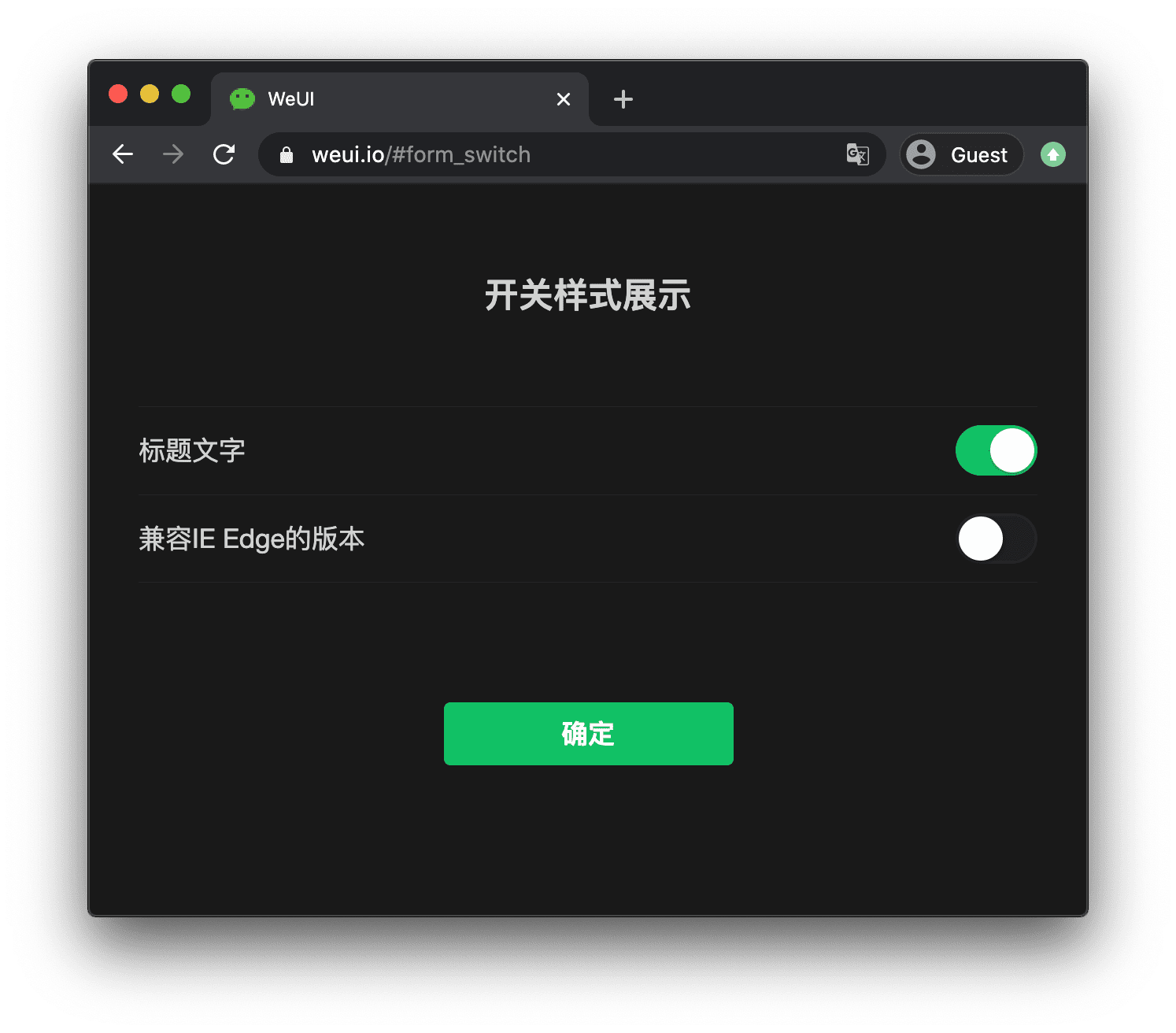
Omi
Omi 是一个自称的前端跨框架框架(GitHub 上的开源项目)。它将 Web Components、JSX、Virtual DOM、函数式风格、observer 或 Proxy 合并到一个框架中,该框架体积小、性能高。其目标是让开发者编写一次组件,并在任何地方使用它们,例如 Omi、React、Preact、Vue.js 或 Angular。编写 Omi 组件非常直观,几乎没有样板代码。
import { render, WeElement, define } from "omi";
define("my-counter", class extends WeElement {
data = {
count: 1,
};
static css = `
span{
color: red;
}`;
sub = () => {
this.data.count--;
this.update();
};
add = () => {
this.data.count++;
this.update();
};
render() {
return (
<div>
<button onClick={this.sub}>-</button>
<span>{this.data.count}</span>
<button onClick={this.add}>+</button>
</div>
);
}
});
render(<my-counter />, "body");
Omiu
Omiu 是一个基于 Omi 开发的跨框架 UI 组件库(GitHub 上的开源项目),它输出标准 web 组件的自定义元素。
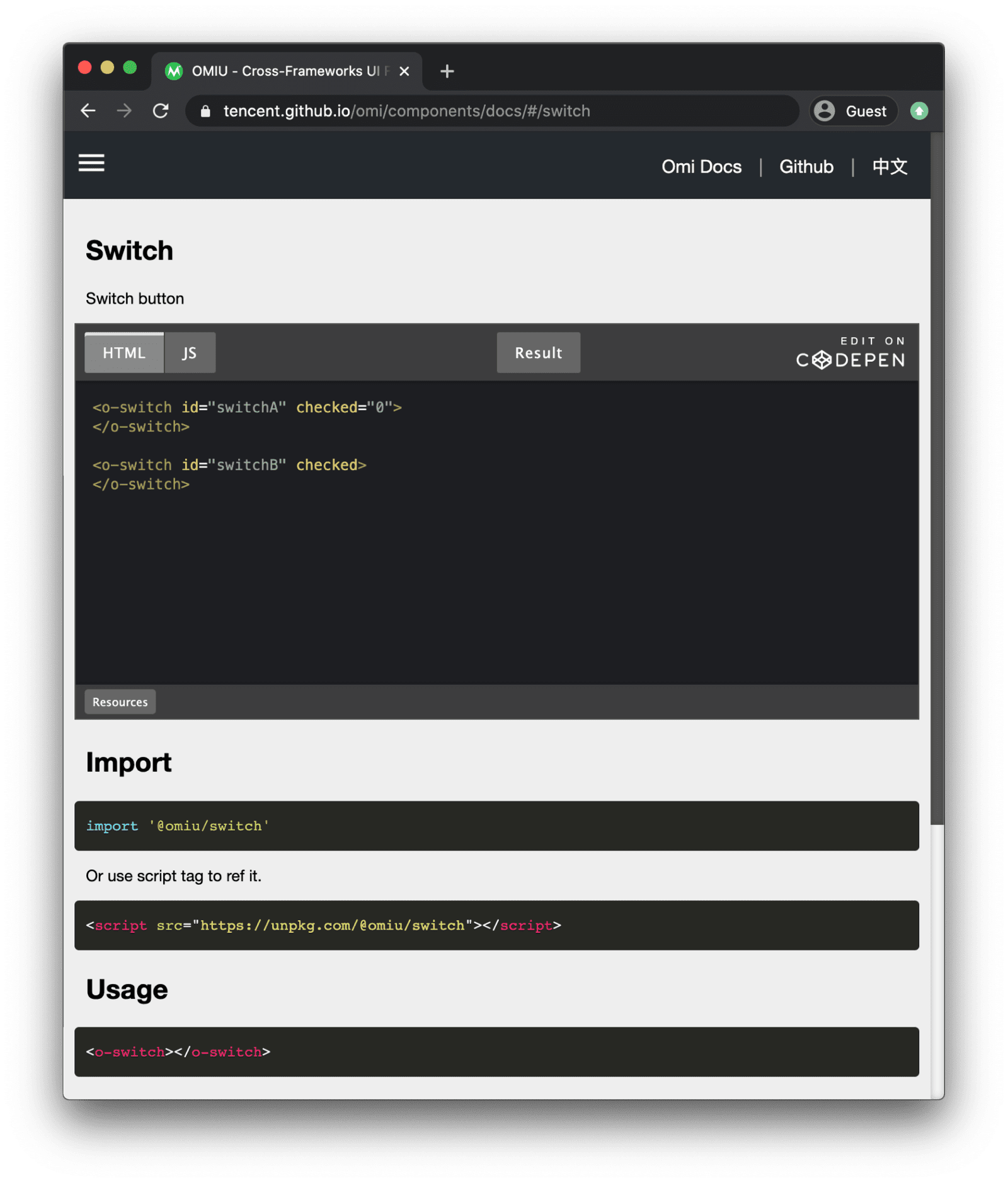
WePY
WePY 是一个允许小程序支持组件化开发的框架。通过预编译,开发者可以选择自己喜欢的开发风格来开发小程序。该框架的详细优化以及 Promise 和 async 函数的引入,都使小程序项目的开发更加轻松高效。同时,WePY 也是一个不断发展的框架,它在很大程度上吸收了一些优化的前端工具和框架设计概念和思想,其中大部分来自 Vue.js。
<style lang="less">
@color: #4d926f;
.num {
color: @color;
}
</style>
<template>
<div class="container">
<div class="num" @tap="num++">{{num}}</div>
<custom-component></custom-component>
<vendor-component></vendor-component>
<div>{{text}}</div>
<input v-model="text" />
</div>
</template>
<config>
{ usingComponents: { customComponent: '@/components/customComponent', vendorComponent:
'module:vendorComponent' } }
</config>
<script>
import wepy from "@wepy/core";
wepy.page({
data: {
num: 0,
text: "Hello World",
},
});
</script>
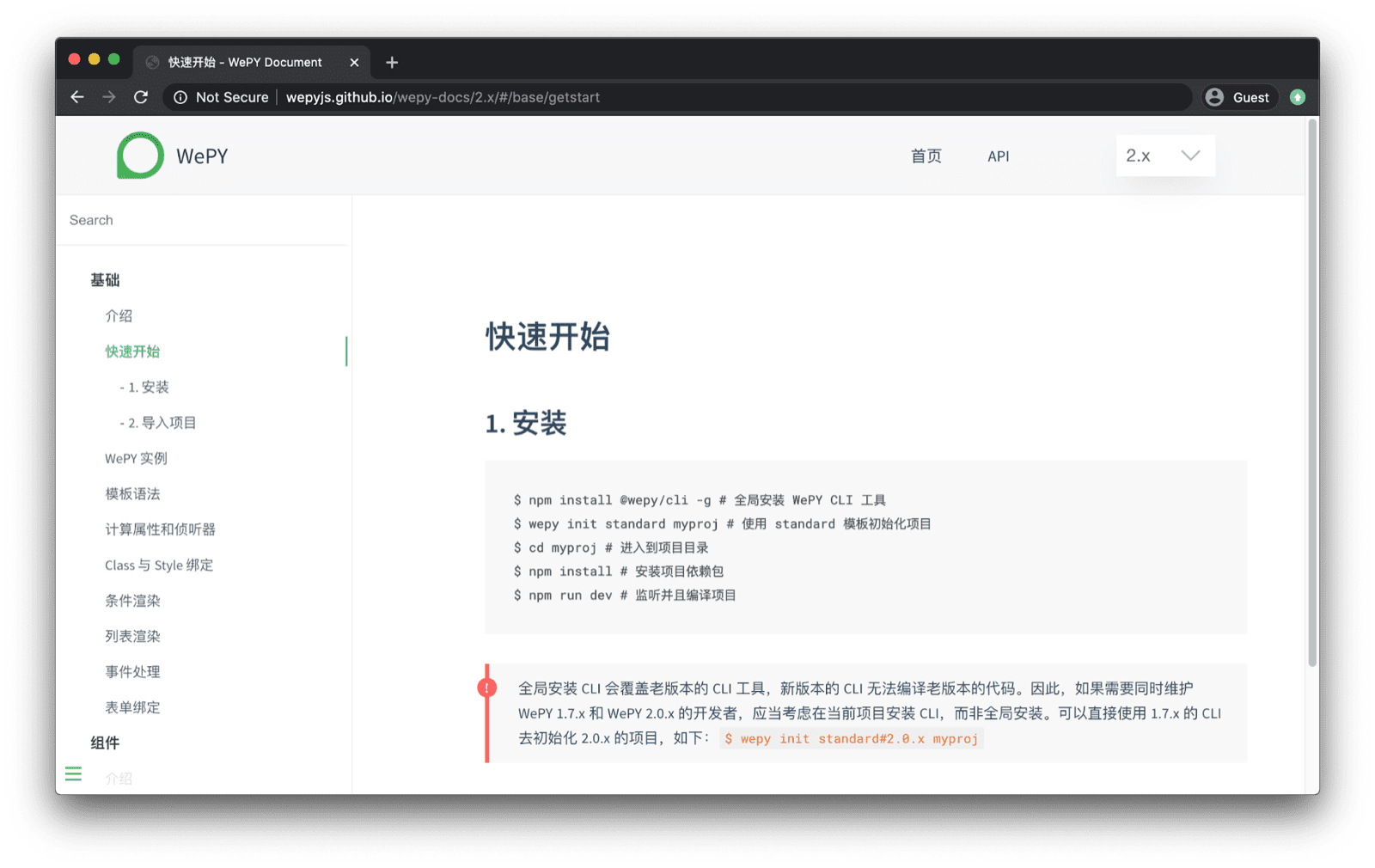
vConsole
vConsole 项目为移动网页提供了一个轻量级、可扩展的前端开发者工具。它提供了一个类似 DevTools 的调试器,可以直接注入到 web 应用和小程序中。一个 演示 展示了各种可能性。vConsole 包括日志、系统、网络、元素和存储的选项卡。
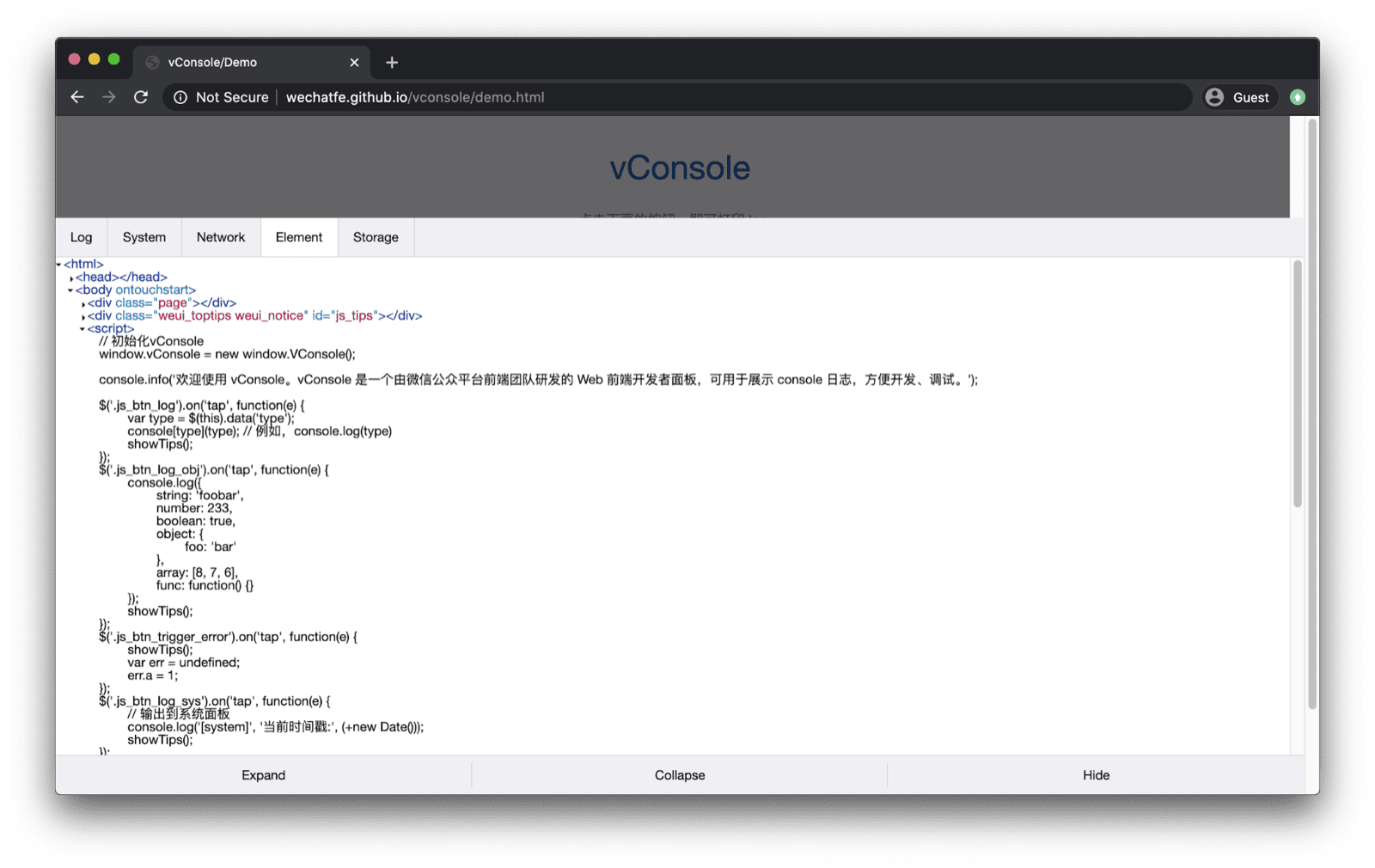
weweb
weweb 项目(GitHub 上的开源项目)是 Hera 小程序框架的底层前端框架,该框架声称与微信小程序的语法兼容,因此您可以以小程序的方式编写 web 应用程序。该文档承诺,如果您已经有一个小程序,则可以借助 weweb 在浏览器中运行它。在我的实验中,这对于当前的小程序来说并不可靠,很可能是因为该项目最近没有更新,导致其编译器错过了微信平台中的更改。
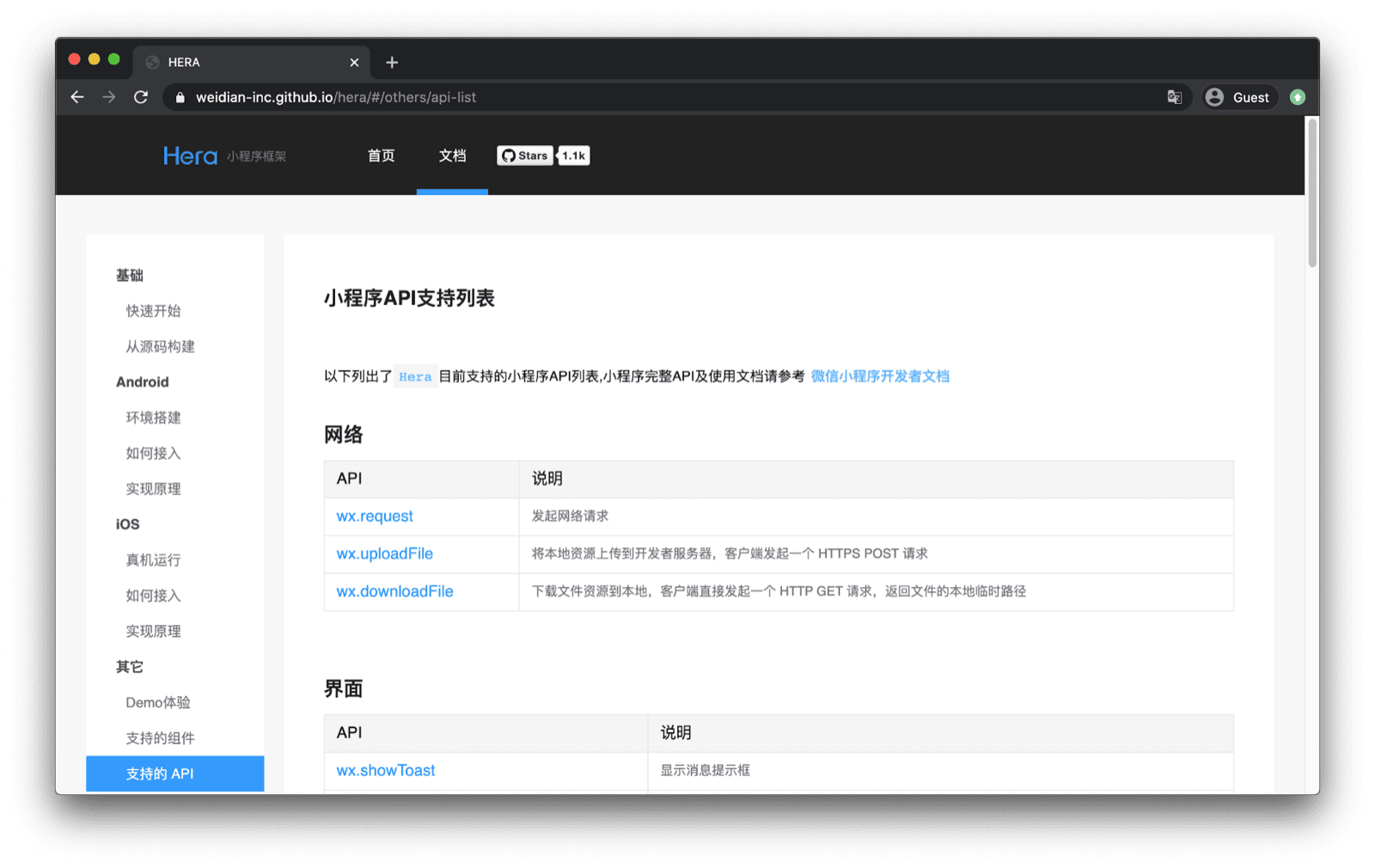
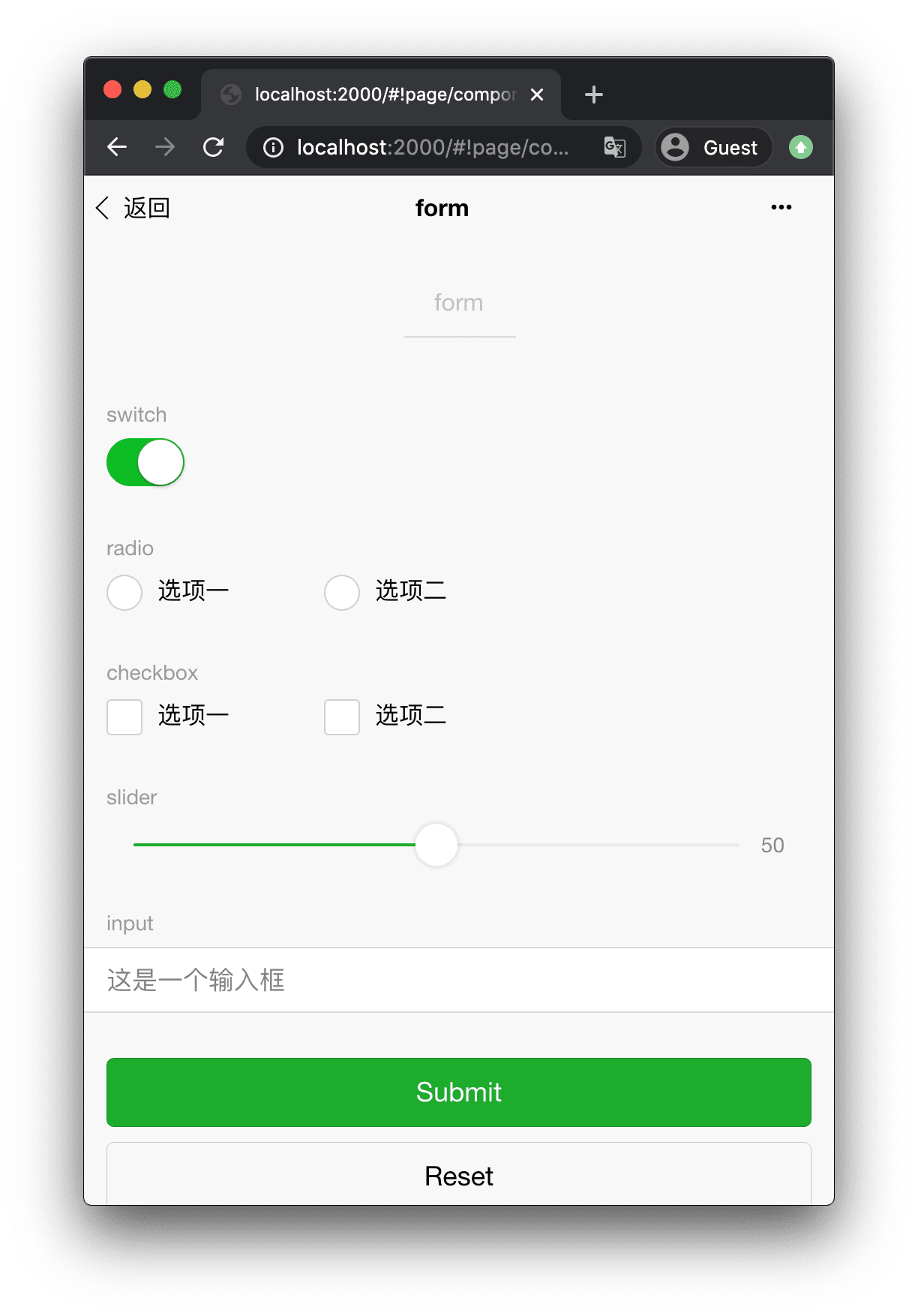
致谢
本文由 Joe Medley、Kayce Basques、Milica Mihajlija、Alan Kent 和 Keith Gu 审阅。